Hello Jupyter Notebook¶
[3]:
print('hello suesser')
hello suesser
[1]:
for i in range(5):
print(i)
0
1
2
3
4
BlahBlah¶
[217]:
class Person:
def __init__(self, firstname, lastname, svnr):
print('Person: self:', id(self))
self.firstname = firstname
self.lastname = lastname
self.svnr = svnr
[218]:
joerg = Person('Joerg', 'Faschingbauer', '1037190666')
print('joerg:', id(joerg))
caro = Person('Caro', 'Faschingbauer', '1234250497')
Person: self: 140616191009600
joerg: 140616191009600
Person: self: 140616191012720
[7]:
type(joerg)
[7]:
__main__.Person
[8]:
joerg.firstname
[8]:
'Joerg'
[9]:
class Person:
pass
[10]:
type(joerg)
[10]:
__main__.Person
[11]:
joerg.firstname
[11]:
'Joerg'
[12]:
nothing = Person()
[15]:
try:
nothing.firstname
except Exception as e:
print(type(e), e)
<class 'AttributeError'> 'Person' object has no attribute 'firstname'
[16]:
type(joerg)
[16]:
__main__.Person
[21]:
i = 666
[19]:
type(i)
[19]:
int
[20]:
type(Person)
[20]:
type
[22]:
i = int('666')
i
[22]:
666
[24]:
s = 'abc'
type(s)
[24]:
str
[27]:
s = str(42)
print(repr(s))
'42'
Syntax etc¶
Docstrings¶
[29]:
def add(a, b):
'''
This extremely complex algorithm uses an approximation to ...
... and takes parameters a, b ...
The value of the function call expression is the sum of the parameters a and b.
'''
return a+b
[30]:
s = add(1, 2)
s
[30]:
3
[31]:
add
[31]:
<function __main__.add(a, b)>
[32]:
add.__doc__
[32]:
'\n This extremely complex algorithm uses an approximation to ...\n ... and takes parameters a, b ...\n The value of the function call expression is the sum of the parameters a and b.\n '
[34]:
help(add)
Help on function add in module __main__:
add(a, b)
This extremely complex algorithm uses an approximation to ...
... and takes parameters a, b ...
The value of the function call expression is the sum of the parameters a and b.
Variables¶
[35]:
a = 42
[37]:
type(a)
[37]:
int
[39]:
id(a)
[39]:
140116274372176
[42]:
hex(id(a))
[42]:
'0x7f6f5cc2de50'
[43]:
b = a
[44]:
hex(id(b))
[44]:
'0x7f6f5cc2de50'
[45]:
a += 7
[46]:
hex(id(a))
[46]:
'0x7f6f5cc2df30'
Datatypes¶
[51]:
i = 2**64 - 1
[52]:
i
[52]:
18446744073709551615
[54]:
i += 1
[55]:
i
[55]:
18446744073709551617
[56]:
2**100
[56]:
1267650600228229401496703205376
[57]:
2**100000
[57]:
9990020930143845079440327643300335909804291390541816917715292738631458324642573483274873313324496504031643944455558549300187996607656176562908471354247492875198889629873671093246350427373112479265800278531241088737085605287228390164568691026850675923517914697052857644696801524832345475543250292786520806957770971741102232042976351205330777996897925116619870771785775955521720081320295204617949229259295623920965797873558158667525495797313144806249260261837941305080582686031535134178739622834990886357758062104606636372130587795322344972010808486369541401835851359858035603574021872908155566580607186461268972839794621842267579349638893357247588761959137656762411125020708704870465179396398710109200363934745618090601613377898560296863598558024761448933047052222860131377095958357319485898496404572383875170702242332633436894423297381877733153286944217936125301907868903603663283161502726139934152804071171914923903341874935394455896301292197256417717233543544751552379310892268182402452755752094704642185943862865632744231332084742221551493315002717750064228826211822549349600557457334964678483269180951895955769174509673224417740432840455882109137905375646772139976621785265057169854834562487518322383250318645505472114369934167981678170255122812978065194806295405339154657479941297499190348507544336414505631657396006693382427316434039580121280260984212247514207834712224831410304068603719640161855741656439472253464945249700314509890093162268952744428705476425472253167514521182231455388374308232642200633025137533129365164341725206256155311794738619142904761445654927128418175183531327052975495370561438239573227939673030106077456848477427832195349227983836436163764742969545906672369124136325932123335643135894465219101882123829740907916386023235450959388766736403229577993901152154448003637215069115591111996001530589107729421032230424262035693493216052927569625858445822354594645276923108197305806280326516736449343761732409753342333289730282959173569273013286423311759605230495171677033163709522256952460402143387655197644016528148022348331881097559421960476479388520198541017348985948511005469246617234143135309938405923268953586538886974427008607028635502085562029549352480050796521564919683265106744100967822951954161617717542997520009887307377876210685890770969411610438028623950445323789591870760289260393489826100774887672852918106468489143893649064784591211612193300707900537059042188012856559403699070888032966871611655961232331998310923225082866180321880439447572986762096935819784385927969250123326935194693207724335527365566248223787833888074999276831633440318604463618703789784313032843823470410944306591471928341190975185239212327674384990561563688432939039442002617530976850605132937101449086396141620556053547335569926700941375271829142407234267937565069765567475934101310225342830080409079587329544213551307302050171598424230760469209732907290141606353960880559202357376885647852240092777111489134492416995607171786298436533978180869474106751111353523711540436599310889697485658800887861974934357929246204051767246012250618404011966289872673803070498361217974484679100747846356194664829224736134115135567179291781968056053726484141128347858241259121954601184412409349782963317042002530418661694962318735860652485410222211869544223788289189712080514575141361964805369723164570564998479537657174548128597406077339158775332355215609435919275199351014222246963017013717419337504919295363295101115292951836282819191821651676455946515828048984256116748150367805267878662716999649296949377045794876146628110929982020737013330324451005385378551188803474148198665114579322684900993000236736168555294173442059925371965244997925483159343706343970371809611470323074186985035054722289027174850333368328300281132910841693150457389933183934593292994942796015309756118708918929528449074243284767006243171171622731766606796101967802204564589015899524704741001158110963633731329388356868949408759334176909387806398584647300588928175998844477486130063153068760070084837267527789777356830042778902772105683833021470279728595336332110564064263909724579949686162908019604141753935768876587992428549912151737924270343248648414247456838889541893241450987505759403013249697541696955330296880219304874163501097920036210238768275176369980977614979636096704348140124130683576879904997436596296495705459524735382000363770324894982103331332913562315169854410415317054193928234723398848453552173203688088312100943941434938282203549650281530751087098604681224802973825631244989331965296202372608586509050307993308652001231671915182765742095689513136184095412121473786311042897717861448158316965848766949554826252504961227044714712229620274682362909803877469376987358942125441792355298387479830450253909788733469732603097544156474805473732732767248652759034995336354126953900458854988683574927864615252040800490114785892289085443353996994780867471613519785838571456421583171193004117989440790268346357550339888086725127883577297626499213827436573992927302238792576924232785487201297255386071968303782483063725899808484638503828356258403917311872694381464553651690062530023217591343084755215901475299149215296944362366910833233693767993138209275870024246238331218236715236772098417187703860172308522448043176333602759733161201262248323085329288986154559221427378507410978822244729512663572225567169779409767341543017289268332635077451210167869121334465680739797372711461919299938118178827541421792926883790285430909942441260511945849237909966329550263865701114884142266162969810073652710928504579470861508094054577797864301504899958634164700528220562786008864025709432444254044034243140203812074857537999016066465520986980790589347320243050635907363821521280600041827529325485247927904235727598574209554632363830932428250711518801775633739811523761994686263270550635099851254333875594601540900862014293625673738331693082328854327001487476635118830885173775268819526360165345900556160767713453617655450974424979076063906093300028416964847594027046669468486593636425428625241644836652173922586528474244952363302305311413449332339822336551611431469131900170488226836525916399723912626616140205707996727383529597479125488961419287261259757561701592645823541151922177253919651034344793680369057003813056557866311011476313189571556336518727757991908862890765494952019474922148851417079252352394293801701149485239005844358329748769279941586384640877265901749104933238853465429979253900561311562288241147192158137210120267399648622831610430287268739840335142120299516610846193164688075944526965248570070554452152547493450434852917987512185973647190461515413582582139040172118295702327537027389787793506904044938553587650503557155872873201596885061331145477101575699375441097493374115991199114962726801718038950907803041184400075585468560976965669584325627283327416418044590727844680051360774154288412712456353383625469068936430902068216750459819321744513362913853983154560610459692604508787700304184579153478291725762810632722108035826060904572460619204237580363147200158749075361633785243462298769917887808671453928846572417223504887766803869453474588831907597355292800709241471370696647029530700507083091412492771404776193459007315206233634226128137074504162520473449597415678882003845446774388950379192344594171245510231738995030348421937088083329709108176561010708693158020695060096428352046647333361163476664106311247065173802510599409266908984046663298613648854871230659903565772327667696057187057276814394932559371368029375974604116075641599919402266794230681485723361363592903676841480358328093127506801111571615062761556607158236612268544268330274725849294875852089790850962835235527978491475563744318483993474633300330972497012808415900969455190375849945750379465019166009861502794606130794726898507849610303884846035423392175449505876157130344700415823080225786693300512126831846009510203543174323783292176865976076275412421892808138872880175813109296020150746331979561488146333412674896256883784351178477592660577212734269328382384711746083782209939646612308343952169576581065423771981899573840430315930973215059901371218399762585055435459516340055149080565627330475362528926945020226163130902420795006258931367813005222140742964756194053782182452833097021554210929638693005460011927178302761563505715735405672652524175925436371863471836292012162456662093642074605500842449347289830619506077570528754845277680661218358066130291463288932240701043887535007851997159198390084654596996197138399723495863749806582439384615049184048485819193560667125968018574877819561104335238420873417743385185735663101292757409280586840011804854994149478736882949368786637202682607198707656286436753775709560349718397405565505269425218354301348910785234517795519757516484711545928466003754558485470994737493796615841040414239875763335201795518644856632201598556341934286668912522153446348791218159622744525372314219184738770596659942181275403613660438538829201810204850917717791485256026242529802492309229562177062770027659288158473994804255067730903420043491632913588644627415318468517462580180901314477358637486528221274450661883667873545037139535563260349778209992416559111602097437491432360787879331015052417047437823553506205617017572175387061751192919715660363028302343819584946594328460482931960515124867123604625653903565173322856758210937541222674223847046664733620292824834065137814475367747671882220098389682019784216724015491253360436437847479770633657905418133523010804559958547379685864708937791659340223795537045273849435441105983887969741143051069401271065628507537039823308867819868298171415185218271493613110963984021912448323423901392553811725954153209435002954807640291982765741514042956666953177304003358701503703497424897898108939453026976878231557938158928996868766367603579055322794822757659104812835219745724022347569914650240636730492833286151875049129873457930874999488048681250802904606446223569562767964898914869924201946458521355165709887118378290437174375625282606140534611987395334677500936625746765638459629521872262777473480491233965194281353725068660782076683862565487279038020486778099991754380815789820825255566234983933217491493864966284116889874665005414748264599972752003370084542592544301190399041231752771993767799847551279448012913842034323154888137932524887172099381195722163148101670274877379161830968937348720168944903299658932511996504109653674618914861599481632040891930577238630396311858213341337110096389113836596895914715370925073998461682046426447290788976525593505136546978364603183820619560578517561504972661817649030304982138534738696212234626114043035600967042547012317360449724623287452575151198771801585742829389025650825988275495110865424704218337264023078045681651420517807418196096401513461760794362769612228126118610912766814880500950963889032877710837651051900076128058473969258768737937306664751387942217354694021157675557568970168734104342446525522568974329716152742558110503495045718931752447070410307760830365536714180388723602948872805590752711115590794756926903978519601939790311768070356801944936106850640568519290645048685535628256787225734544146565541187816717729850612874044620890718502108518025052924590359814117522720320552642597751984410742492179242039080014606225999422109717176118746845802673724801365603866909971071347255859723217027554055085082090418987534829222004178998475030519537179062001509333023023881806519182405550818672164711702307529922652228033820404113386625335815042934115143980939986416365633923620673874259342713444701242702722227197573203194489407856355511639619115985907995399083680129468810771595938084908111251938016414866250141095286680914828503123938960997659175977315432797173945762560365023587931559926170852315074247849814256564693008105061976397395591733545472917526739598790117477449217745771908169489543790314578152667389689410604588351445026130645637237687631129964576699475767340673583537218704935177320214779403972566532581731659202199752942824432778102107532160580104432121067208273876100778332426569624765621063126975491546224343978061253999313893915782008560011171319773443130412998215626985098895722778159524505640435534979855872234521991778419564106622122049039017886737997905270552302412002780864726282517525092332555378737792434915926182736151242592242725872699844014132567954640475742451126102857394193479716383187138370722782422619384021010896271281685732287764210298708895557148397743497418109849633633910397778254225179400022143485886207532122646613614487518735142494469575836744785028013193033901949738716163113800864093408529297729741462836142201120573027427309566658849884965134295188287937016147499504685185116851709758146686994243673140036992381232483920618628663037402351333907771907455224248515748726136075048020960976567862025232356273025557054386729189255571672396871991669651834736987440295942395220863481341484029838939485593327256627318194137735451891544384960966451285561476772564932516923822003229733483331726306200059192067441136913243720403578098676440894672367334549903905283682411422011886949265324531399323764100563996744273693606586851243936737155349696358970470706246743064681511525580772367129358691546677179280674603197443660235664811911175324239792271603213932690900431433572511935591785787438931910836722219749594385217682817205537433987939372420698422586002026702204502341484432231418159837133223467338185995720215212999066855318593765188122815644349842897804044474253173187198249880219056124756835053121936684091113613451740380400041281474527453687530122741226292549431008458868082627169312891414237466973215688306962596297824286828479758198382340345222708757345393897126910174835179971320922911746435455023926629385455742630840473245989374307836875864087914312319228307304975362481708579849870550314357368660453504099679685389802578477910419231052505144696885248726344365369453921372194715998156624454330562798282777349584666757018969900985110164491047993602922961645132995224271404908186948080818144765441476070834943179921347693205775493727581146799477819807044909830887802418354528095576268178221596410712216509995196961932368766959924336939018675830477951348564967496643006978548120861013029026482640402316621018258604172734501970838570222765188590393123627245232006535983907604127898007628220791246163222713051906678029644273228763460060289213316094057396003523608149487855784819923604033709243920895785100953666245538047118419834068589948934026518132741951749125192568729824877982938351710224389886834755562218214866075518628420508441240855088408499405226548535776678864444680504301285988469915501692621173049550268365498340637943341584797390666973271817567111123445212701138287733170703648843560692535012857745637166012927846194135756491508269323010063559087991831480179606664893606864875569154700860602703909640090514609360513037280984989976472934205971076104365706670369636731086616773064361333184164332104073479210775688703754193241101764488071673304176268034528127946729244452919161882095335945667158450941476301537032588109254921620602423998383939639570864268315557737923542830477169053850721939927266830567227442913752680237178175908437269780708099690192695002592421020485193953805155158663266418230452129371046840218806665165231438597498081621420148051355131865453014871298249938624272154345372391802215126115854861975398983110888196358875565793593310597895992053240439684590862193232015232257668969509415398393721308747247732944493053757577694362680328316055060353219685001207195191671426063364056179006218671604638684249067247572855764071631397824639188693789635247708232195940400450829593853651517642510129335911478337995658501766175785429966837417247838838645892319920041196390925354226074199412181950196251598137076467085022479201649174056794994024967593003129957099742026595785390010668925864718839026058417582396497109522944729874183273952292247592156943695682037477731049514128818431210317476600507328613045698141876738705835589680568812062901672389707603039549708273418484069037215179862266581929555420755695654139976814235027490594662592770986044588793675562143709648705744665319814772892177290937799834952769995145061157204394128687121538756842568036622321369508041915737469009917048039885947260486258684449762362318724085339500204989295963618739437408918888568903691128987832325892601331155260901131911962536529384643993465666490271108522834470184768391573623895239732781158399355178022076177450755929138381468526577309152290502509145241134006895661073761320484545645543610238773087594308408699782711315425513472058939339453013548135244176754131385012770024156261936169649709944156933541666590935759784775946468953923516229723673403650805886186209888123677098892944769654360324668874429740130365009770291603536426502844618046128137324006034947889707723262364927515545013731797277615272236008596598736693841467685030166103516988390995570659331713219574003251046303604196295276316972893990945091279545361627593486818455830168634280653205028729105026906643726170661778439311154737149759096116144493812106746037251452616271615212935043141124155849847574091878458410177325030553207237661933970254488335435069113577757765584551136714634629223114497809546405079026755854160954260557783406467356189398503160303046687522579708387993298769182986822970667356246647939653365454544268386404322650296028881298557242198187208040207296077496128886166773288838620609977925416822156713703667391333322086520871904473048593017466793578173830832406459585018177267447690376523178740142636502172093102619263046542112708094769682392207383797482057034793196525139221370199877543931178601238198924707047629587501516299912892933069025343575488251454948469328322898202956506903308470482517744131166464774750723477861518055343466858040987132273453081836794564364430155246274158100731069323080734901590517008753654169600998911569598454702926629374049393677685285723039754691181021469267219333846563053673846537824970049506209403506606244584412627902384242785280551599253188262786271531989567165398385428909311577122438618119724985644233907558812574859217687149721341833317289714977694982117227337669878361212788465289349470676995146785835955074121568375032937822352378665103266923449887690619794116574936418347914823983988002067649967289214983486424468737742286438563064425223461210639952418146166111391586408089740413945879849036682695488197808766021959964846771145698159367567904768112047055740602351237499012258567357965107013251562237843754994747236883821060645969480067316032381351192535148322216761442411144695361182838761115795911310362420741510826322048374540404146990479647073113428368300158247350712922331119789013179701651764033036649606749518628656836032556353205617386602749327417799524243899880581322620681297935615821202736455540414428705857131835238951742471239050758919353490570268302812810704750603181709274434806504843422134152201579626776393642698168851485545206897802036257496043584180735523092410264794436423951474855853980259367017205588767985309971043290404884467541701918912275745752402121744890326681160647837985004639864193645792082105266057503921195311457592621400830660301768120178560328650220171413811642155986702637531155554821958388905393544592180587272433749899242041542816666821846374863337522729077994208960545264863862516411718049112903451378140021506899966762080732651380427792460930762263446994159193223142565029996403134383564087766689537759857473403120477091886596355579944643688205981242040477802943803932459722207541739589596692034052161680837588921636048584789929663903107349945667027830610483130862872680661015300745521669893857383782690975026078691245077096697355744971063878379083608563324774011426604588797712843335089341752120843133549155805945217503766728136818989701075795034975931147113851177529084708382646930943663837851488798723688771534770333456570254004964039987760745951276145936694695508877796318400093229973736770518512573233230127134210937068680950264362225510872877141635601716276267040168996816287213326422268377855327887822310204539762272583014902609710931194995685950834598509258803363019551891846212990914920155838298607568190737375958573348261713794703592503972126314316192159571451081744034114847904386601284244643362857392442316767411036417557776991886719910511456902002905771699177497748359489810115650557253501242519595931483440417116222205504589671636754478042893488385500275211497526246421122010774862333476246182576597178403980436617669560567049936789350370893174617560282074679058110806280348893136768773133234721178541038289213080580369302853910384704528018344058313252744129928355732704028220901951560054449764635932065083760864473989692709381120504251266930834839999956860216356148940167442564078469350152730245749435722205009403680810321136898946443062041659878360406811331217133504960504944165472883926378607868385731515366373416312566122603235977742473940324793478740770352725436532455308438250865693864330020957120719122826689237609273524467576647981797622831709248187143189474449928329051087066866263901462754600186683583622062445553828867143621415773638244852570664653423002003386087185683387095282564231892337305827467825102905239214464117590700082862874915647212962223100149842773910585262712095702633425139069275541249925826898755913048489059083665047386569005980726512682643809800080768716889749767005747228285165430935469423701296901225200041775975278099341399149455675655415791289014141807180970818010668626000334746783492116354330741766556197865412944331579610856733907210970815247694360208231077377319255935591919361509992241851869762511821971598449396422923222586939583205849537600173388242916813257003198490942805178528782842560021549233235032941872842601109623432061485547579038802849228139397130979180562447703494299530585909415031237400213445431607049538809117870571045686211621069655023028886617060314580222884344906879404890744067164557565160596397488164744575409400891911083945126233990453364772541741331330821153758983077390402549739751620825837898384996200874749622331436354553599710472896396262914675542281735624665804212007275401273982269651802596130334329792865330063654175676003369688243926391280713123038760076039957285658333390838592534249337153070457471482743551037417147024006867419141874398230449202772009682282474640802779068104741602882613440803256607530230172031981070796893051954145239327661783905828943932146148894821695918579498502263858318704473290299790473193753803543920285706350672484245008920689712995308213747335255792982565803469779504974053329506220584586976782376547358092670753891736652401577525890202354883227480678961328466821865972869785592672826822656457577958670571097728396605101516387163615321918203652729897586807786443044896628670393188486921931839182927172554503767733076856308657600665342622254535057629030862718937364327448083558928220102374951534665262857504222950995446014428801859061443379679142717413278286975998259993819084477469775220567898395378570154609157695006128689610825519373486612568225010585752375050450503641627804406676905130564807191014132285063482665726828511933059433717373899623654715534816591630086838520905491060194596758223701911513581142071521220673521948777069521608531717477580542554138038978873716058241771904252401056370661792996395808706717083872950238057238547610495061732689587948240319569499000594593719849004104550034415014066154526994609094441391439071866309009249691812468813088126242358787204856089608234037677437509496768451058059579977189006605354903359961784245121096144877063187466910292356627771165203522647016166296488083808997887687769308220856530068153940856748041349469491870704992660210066455819832886130678021460369893174499939107231230405088342485383796742208847060385334943928147519685791292174441917386398739155908915859310750449419969425856920503891277550092560029225566028245733054164370482965351532695757311986033153861767127072322962670122480612322195488891848040149357757131302308897501348573600357134307046628157178720021578705983302856401851403674168523579202306440644159448586566246905477964086572462611834063213025126230896949713642334585419351128373132157232101577687884147986752364441711298472354763142715850293434746434127230225735792097083439910498394019789979870351837786010778667226102241684498686398874227580631272581474396676719404444290071284914286251867085092358453277091441099575357659812178523756848934429670368686181229928033433456696493460770903592231804072849827640955873854491455416480621098619090449331761288966135325413062313991971462332768487224184119088841054819270878948939590681778579588918587881426099241767753862382406002451821149035160124492893940578494055432173589779503867792448614769448734671863583817296627073341404027631029821562303142157743379294616649374361560459036060298581224576085252700570061877609582745974967405315882162695982851143006320897730473133461172142945805407178876223011462558510520930761771493278603541262719880522826864107055049364370555816945830840650960447321868266858216903784868032280479864021706315653687489290467679015864709379478762373013822026373788822827564163725872670921602434077279298565679825234946428376162918717556993454693724759744644374586123577768842647346924365909558553162571564807969034052671504760754742676960038255814034354716520834598864538432593908154392918939089896109083521992519053530398359081516289247571043354786424954980741388745386023113329591390636006694539437438690759589121288758007804082911975207095278133335550232776761783391381794105109412999680430103899475051243232331431120663598726618610084717254696908726637456858466646162093223427680684601718516702427671125913080059583053658180035991214447685286534985397013876080817020918472863351368783745251292936294373137797794168163130983688833624592488270042533275310174037401858155192663147588032450316349828944090941459435709839331454077937638533884824056964583661906580362312059760037127664830995125859310214290237306052000883415749724405028863402500028747055233688541068593389039662379913776485715772093749681055718277781429659244251666703110135820625316518906936462663613376783677001574909561444962828205690820330013121289873007855206280915035880334071746233511142838096550651818271177825964147342275885803162473648253508879276299074813242692461714169521068548818275361612786955216412383263246164034602771053052217127416909831714425842754359325193708367920524870186212708574531518197079797624156322369627530303625010057401503185241771381716916959066550480494267473696656051819527729740440782487416353087074803447126307550453018768734511631260002571765708294113447159058326859464022975489061868569715529325333679192904509181338989039531226114077604146341395120253199068720690450654317360799352434791082039695478061926453254644053124586362026016156957888326419910345519741792140396061817459467332211367113051675844970722080638249309285348257747082754939860608508486386549912912220391895379332321814344998458680557267915553525212113907723389037979427485209309504641599639196053296030292739274663906234190084882219800040754390250718430168480866157009022053158273857417267902310193566064243660834080202786392263652453395504706244108957791049298908397097715806857036527872932846771952274875201321856644284239116502005786314083266606564469023548865452436995428173054383481532525156311603438941440612599662264959878769003712412406305072713049361060777519351766684350979095112470377728527045181653556285621656761979382960837252084759203181114384404005114613990326273362146150667851880079327563486444803726963278475818453468509565365380723807736793526242145533088833679727074192838872685484977387306670007551965761431583941102042492623133242770270821710047137152167948872468741189259805623910487138975034203376673344989060052111727844670530521327425332189734978888307943626099125569741350839434061750712876445222039557455150019442996232739317091487905148812821582894579949748623433452865460274945133477267345362799684706610937707688048379567860499887327287273158785195505216946986571759372875984514994087366735699690322212619073372131984972873714823922056361158276719293687517950957314775347729780073870450969204201405641649670458849057466793682155521901095027966023590200629563426621697556166261956721403439803621918243177242110484823901622121433246394511832190737036276362556947535372619436126996645342229589006736952014870393865015087074414735220220770411070088328030731545041085721762448600324574529642445801550887535581445128918173686346116567111050151109714760544361537920207546115073529167935359803746233158035402507293585422134950239655004818456256327794930441926282941363007224150758144477688955569616025741423859274415740477217643474948663183182648210083785749080222199122144694117400270856159095104885845967392332602301843740328702808569782311182460392642129024729192535226787567897868564600570545108945374190649371558894253329703486982693386634574807801078719254129784907155276683405362407228975018016417486695226639468897799829747034414846605232977531914266638473906810541671168066131262918834764800639654762345192540580400257727950859061817326000188356677576249635084512266410557551645134470262083485480776173701923626406384831371842087477223094467978661221649689842322789248762605167898686475739012260361067924661728390016267344059903224026536343550301552983264519865881303942586617734987280231892544278016113318354276103258002393942607858756949485119300715853929843163967575531352484221274814864027297307155457109423432471856970523635672286551516265672389248080659279448953078708153606666741314598260798811278754656313007785743484189610809499119436037561075326359414143476882494692530787790693275290895909945065272071979419682741840819830567497688086236460819345623637702467815073133466197923119939117195979352833117496820500577299938566476984260815518940866776320855483159836188850448339911351505132909703990088919063133375804094886955895465912648104534621430295733375992898959329664444686900648674531661878821297194146191914132978673375283702995190706179924178140249356285006536273771636355569720003246996130665282451695167273161831393867558791278264933758040011059211372270839123518459709107480669852696080909181872978501574064544548264471078633386599113188810137746318984496745989011540334259315347740082110734818471521253374507271538131048296612979081477663269791394570357390537422769779633811568396223208402597025155304734389883109376
[58]:
3*4+1
[58]:
13
[60]:
(3*4)+1
[60]:
13
[62]:
3*(4+1)
[62]:
15
[63]:
9%5
[63]:
4
[64]:
4*9%5
[64]:
1
[65]:
(4*9)%5
[65]:
1
Compound Datatypes¶
List¶
[66]:
l = [1, 2.0, 'drei']
l
[66]:
[1, 2.0, 'drei']
[67]:
l.append(4)
[68]:
l
[68]:
[1, 2.0, 'drei', 4]
[69]:
l.extend([5, 6])
l
[69]:
[1, 2.0, 'drei', 4, 5, 6]
[71]:
l += [7, 8]
l
[71]:
[1, 2.0, 'drei', 4, 5, 6, 7, 8, 7, 8]
[72]:
l1 = [100, 200]
l2 = l + l1
l2
[72]:
[1, 2.0, 'drei', 4, 5, 6, 7, 8, 7, 8, 100, 200]
[75]:
l
[75]:
[1, 2.0, 'drei', 4, 5, 6, 7, 8, 7, 8]
[76]:
l1
[76]:
[100, 200]
[77]:
for element in l:
print(element)
1
2.0
drei
4
5
6
7
8
7
8
[79]:
8 in l
[79]:
True
[80]:
666 in l
[80]:
False
Mutability¶
[81]:
l1 = [1,2,3,4]
l2 = l1
[82]:
l1.append(5)
l1
[82]:
[1, 2, 3, 4, 5]
[83]:
l2
[83]:
[1, 2, 3, 4, 5]
Removing Elements¶
[118]:
l = [1, 2, 3, 4]
[119]:
del l[2]
l
[119]:
[1, 2, 4]
Tuple¶
[84]:
t = (1, 2, 3, 4)
for element in t:
print(element)
1
2
3
4
[85]:
5 in t
[85]:
False
[87]:
try:
t.append(5)
except Exception as e:
print(type(e), e)
<class 'AttributeError'> 'tuple' object has no attribute 'append'
Dictionary¶
[88]:
translation_table = {
'one': 1,
'two': 2,
}
[89]:
translation = translation_table['one']
[90]:
translation
[90]:
1
[92]:
'one' in translation_table
[92]:
True
[94]:
'three' in translation_table
[94]:
False
[95]:
translation_table['three'] = 3
[96]:
'three' in translation_table
[96]:
True
[98]:
try:
translation_table['four']
except Exception as e:
print(type(e), e)
<class 'KeyError'> 'four'
[100]:
translation_table
[100]:
{'one': 1, 'two': 2, 'three': 3}
[102]:
translation_table['three'] = 3.0
[103]:
translation_table
[103]:
{'one': 1, 'two': 2, 'three': 3.0}
[105]:
multi_dict = {
'one': [1],
'two': [2],
}
multi_dict['one'].append(1.0)
[106]:
multi_dict
[106]:
{'one': [1, 1.0], 'two': [2]}
[110]:
translation_table
[110]:
{'one': 1, 'two': 2, 'three': 3.0}
[108]:
for element in translation_table:
print(element)
one
two
three
[111]:
for element in translation_table.keys():
print(element)
one
two
three
[113]:
for element in translation_table.values():
print(element)
1
2
3.0
[114]:
for element in translation_table.items():
print(element)
('one', 1)
('two', 2)
('three', 3.0)
[115]:
a, b = 1, 2
print(a, b)
1 2
[114]:
for element in translation_table.items():
print(element)
('one', 1)
('two', 2)
('three', 3.0)
[116]:
for element in translation_table.items():
key = element[0]
value = element[1]
print('key:', key, 'value:', value)
key: one value: 1
key: two value: 2
key: three value: 3.0
[117]:
for key, element in translation_table.items():
print('key:', key, 'value:', value)
key: one value: 3.0
key: two value: 3.0
key: three value: 3.0
[120]:
translation_table
[120]:
{'one': 1, 'two': 2, 'three': 3.0}
[121]:
del translation_table['two']
[122]:
translation_table
[122]:
{'one': 1, 'three': 3.0}
Set¶
[123]:
s = {1, 2, 3}
[124]:
3 in s
[124]:
True
[125]:
s.add(4)
[126]:
for element in s:
print(element)
1
2
3
4
[127]:
s.add(1000)
[128]:
for element in s:
print(element)
1
2
3
4
1000
[129]:
s.remove(1000)
s
[129]:
{1, 2, 3, 4}
[130]:
s1 = {1,2, 3, 4}
s2 = {3, 4, 5, 6}
[131]:
s1 + s2
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
/tmp/ipykernel_189565/1743780635.py in <module>
----> 1 s1 + s2
TypeError: unsupported operand type(s) for +: 'set' and 'set'
[132]:
s1 | s2
[132]:
{1, 2, 3, 4, 5, 6}
[133]:
s1 & s2
[133]:
{3, 4}
[134]:
s1 ^ s2
[134]:
{1, 2, 5, 6}
References, (Im)mutability¶
[135]:
l1 = [1, 2, 3, 4, 5]
l2 = l1
[136]:
id(l1)
[136]:
140115753096128
[137]:
id(l2)
[137]:
140115753096128
[138]:
id(l1) == id(l2)
[138]:
True
[139]:
l1 is l2
[139]:
True
[140]:
l3 = [1, 2, 3, 4, 5]
[142]:
l1 == l3
[142]:
True
[143]:
l1 is l3
[143]:
False
[144]:
l1.append(6)
[145]:
l2
[145]:
[1, 2, 3, 4, 5, 6]
[146]:
l3
[146]:
[1, 2, 3, 4, 5]
Copying to be safe?
[147]:
l1 = [1, 2, 3, 4, 5]
[148]:
l2 = l1[:]
[150]:
l1 is l2
[150]:
False
[151]:
l1.append(6)
l1
[151]:
[1, 2, 3, 4, 5, 6]
[152]:
l2
[152]:
[1, 2, 3, 4, 5]
Nested Lists?¶
[153]:
l1 = [1, 2, ['drei', 'vier'], 5, 6]
l2 = l1[:]
[154]:
l1 is not l2
[154]:
True
[155]:
l1[2] is l2[2]
[155]:
True
[156]:
l1[2].append('five')
[157]:
l1
[157]:
[1, 2, ['drei', 'vier', 'five'], 5, 6]
[158]:
l2
[158]:
[1, 2, ['drei', 'vier', 'five'], 5, 6]
while
loops, and else
¶
[8]:
import random
ntries = 0
win = False
while ntries <= 6:
ntries += 1
eyes = random.randrange(1,7)
if eyes == 6:
win = True
break
if win:
print('hooray')
else:
print('lose')
hooray
[10]:
import random
ntries = 1
while ntries <= 6:
ntries += 1
eyes = random.randrange(1,7)
if eyes == 6:
print('hooray')
break
else:
print('lose')
lose
for
loops (and else
)¶
[14]:
import random
for _ in range(6):
eyes = random.randrange(1,7)
if eyes == 6:
print('hooray')
break
else:
print('lose')
hooray
Iteration¶
[18]:
l = [1, 'one', 1.0]
for element in l:
print(element)
1
one
1.0
[25]:
translation_table = {
'one': 1,
'two': 2,
'zwei': 2,
# ...
}
[26]:
for element in translation_table:
print(element)
one
two
zwei
[27]:
for element in translation_table.keys():
print(element)
one
two
zwei
[28]:
for element in translation_table.values():
print(element)
1
2
2
[29]:
for element in translation_table.items():
print(element)
('one', 1)
('two', 2)
('zwei', 2)
[34]:
for element in translation_table.items():
k = element[0]
v = element[1]
print(f'Key: {k}, Value: {v}')
Key: one, Value: 1
Key: two, Value: 2
Key: zwei, Value: 2
[35]:
for element in translation_table.items():
k, v = element
print(f'Key: {k}, Value: {v}')
Key: one, Value: 1
Key: two, Value: 2
Key: zwei, Value: 2
[37]:
for k, v in translation_table.items():
print(f'Key: {k}, Value: {v}')
Key: one, Value: 1
Key: two, Value: 2
Key: zwei, Value: 2
[38]:
s = {1, 2, 3, 4}
4 in s
[38]:
True
[39]:
for element in s:
print(element)
1
2
3
4
The range()
Function, Iterator Protocol¶
[13]:
for element in range(0,6):
print(element)
0
1
2
3
4
5
[41]:
r = range(6)
type(r)
[41]:
range
[42]:
it = iter(r)
[44]:
type(it)
[44]:
range_iterator
[45]:
next(it)
[45]:
0
[46]:
next(it)
[46]:
1
[48]:
next(it)
[48]:
2
[49]:
next(it)
[49]:
3
[50]:
next(it)
[50]:
4
[51]:
next(it)
[51]:
5
[53]:
try:
next(it)
except StopIteration: pass
yield
¶
[79]:
def even(start, end):
print('even: entered')
run = start
while run < end:
if run % 2 == 0:
print('even: yielding')
yield run
print('even: continuing after yield')
run += 1
print('even: terminating')
[60]:
for element in even(2, 10):
print(element)
2
4
6
8
[80]:
e = even(2, 10)
type(e)
[80]:
generator
[81]:
it = iter(e)
[82]:
next(it)
even: entered
even: yielding
[82]:
2
[83]:
next(it)
even: continuing after yield
even: yielding
[83]:
4
[84]:
next(it)
even: continuing after yield
even: yielding
[84]:
6
[85]:
next(it)
even: continuing after yield
even: yielding
[85]:
8
[86]:
try:
next(it)
except StopIteration: pass
even: continuing after yield
even: terminating
Encoding, and String¶
[87]:
joerg = 'Jörg'
[88]:
len(joerg)
[88]:
4
[89]:
type(joerg)
[89]:
str
[90]:
joerg[1]
[90]:
'ö'
[92]:
try:
bytes_joerg = joerg.encode('ascii')
except Exception as e:
print(type(e), e)
<class 'UnicodeEncodeError'> 'ascii' codec can't encode character '\xf6' in position 1: ordinal not in range(128)
[103]:
bytes_joerg_iso_latin_1 = joerg.encode('iso-8859-1')
[104]:
len(bytes_joerg_iso_latin_1)
[104]:
4
[99]:
type(bytes_joerg_iso_latin_1)
[99]:
bytes
[114]:
bytes_joerg_iso_latin_1
[114]:
b'J\xf6rg'
[115]:
bytes_joerg_utf_32 = joerg.encode('utf-32')
[116]:
len(bytes_joerg_utf_32)
[116]:
20
[120]:
bytes_joerg_utf_32
[120]:
b'\xff\xfe\x00\x00J\x00\x00\x00\xf6\x00\x00\x00r\x00\x00\x00g\x00\x00\x00'
[121]:
bytes_joerg_utf_16 = joerg.encode('utf-16')
bytes_joerg_utf_16
[121]:
b'\xff\xfeJ\x00\xf6\x00r\x00g\x00'
[102]:
bytes_joerg_utf_8 = joerg.encode('utf-8')
[106]:
len(bytes_joerg_utf_8)
[106]:
5
[119]:
bytes_joerg_utf_8
[119]:
b'J\xc3\xb6rg'
Send iso_latin_1 to a Russian
[108]:
joerg
[108]:
'Jörg'
[109]:
bytes_joerg_iso_latin_1
[109]:
b'J\xf6rg'
[110]:
bytes_received_by_russian = bytes_joerg_iso_latin_1
[112]:
bytes_received_by_russian
[112]:
b'J\xf6rg'
[113]:
bytes_received_by_russian.decode('iso-8859-5')
[113]:
'Jіrg'
Regular Expression¶
[122]:
line = '2435 ; Jörg ; Faschingbauer'
[125]:
fields = line.split(';')
[126]:
fields
[126]:
['2435 ', ' Jörg ', ' Faschingbauer']
[128]:
number = int(fields[0].strip())
number
[128]:
2435
[130]:
firstname = fields[1].strip()
firstname
[130]:
'Jörg'
[131]:
lastname = fields[2].strip()
lastname
[131]:
'Faschingbauer'
[132]:
import re
[137]:
regex_str = r'^\s*(\d+)\s*;\s*(\S+)\s*;\s*(\S+)\s*$'
line = '2435 ; Jörg ; Faschingbauer'
m = re.match(regex_str, line)
[138]:
m
[138]:
<re.Match object; span=(0, 39), match='2435 ; Jörg ; Faschingbauer'>
[139]:
m.group(1)
[139]:
'2435'
[140]:
m.group(2)
[140]:
'Jörg'
[141]:
m.group(3)
[141]:
'Faschingbauer'
f-Strings (since Python 3.6 !!)¶
[142]:
joerg = 666
caro = 'Caro'
[143]:
formatted = f'Something blah blah {joerg} another thing blah {caro}'
formatted
[143]:
'Something blah blah 666 another thing blah Caro'
[144]:
formatted = f'Something blah blah {joerg:010d} another thing blah {caro}'
formatted
[144]:
'Something blah blah 0000000666 another thing blah Caro'
Miscellaneous String Methods¶
[145]:
s = 'Mississippi'
s.count('ss')
[145]:
2
[146]:
s.isupper()
[146]:
False
[147]:
s.islower()
[147]:
False
[148]:
s.lower()
[148]:
'mississippi'
[149]:
s
[149]:
'Mississippi'
[150]:
s.upper()
[150]:
'MISSISSIPPI'
[152]:
start = s.find('ss')
start
[152]:
2
[153]:
end = s.find('ss', start+1)
end
[153]:
5
[154]:
s[start:end]
[154]:
'ssi'
[155]:
s.find('xxx')
[155]:
-1
[156]:
s.index('ss')
[156]:
2
[159]:
try:
s.index('xxx')
except Exception as e:
print(type(e), e)
<class 'ValueError'> substring not found
strip()
[187]:
line = 'abc \t'
line.rstrip()
[187]:
'abc'
[190]:
line = 'abc\n'
line = line.rstrip('\n')
[191]:
line
[191]:
'abc'
[192]:
len(line)
[192]:
3
[161]:
filename = 'garbage-data.csv'
filename.endswith('.csv')
[161]:
True
[162]:
'666'.isidentifier()
[162]:
False
[163]:
'abc'.isidentifier()
[163]:
True
[164]:
'_'.isidentifier()
[164]:
True
[165]:
'int'.isidentifier()
[165]:
True
[167]:
type(int)
[167]:
type
[168]:
int = 666
type(int)
[168]:
int
[170]:
int
[170]:
666
[171]:
try:
int('666')
except Exception as e:
print(type(e), e)
<class 'TypeError'> 'int' object is not callable
[172]:
type(666)
[172]:
int
[173]:
int = type(666)
int('666')
[173]:
666
WTF??
[180]:
'if'.isidentifier()
[180]:
True
[183]:
'if'.iskeyword()
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
/tmp/ipykernel_4000/3227798639.py in <module>
----> 1 'if'.iskeyword()
AttributeError: 'str' object has no attribute 'iskeyword'
[ ]:
[175]:
line
[175]:
'2435 ; Jörg ; Faschingbauer'
[176]:
line.split(';')
[176]:
['2435 ', ' Jörg ', ' Faschingbauer']
[177]:
line.split(';', 1)
[177]:
['2435 ', ' Jörg ; Faschingbauer']
[179]:
l = ['aaa', 'bbb', 'ccc']
'|'.join(l)
[179]:
'aaa|bbb|ccc'
[202]:
line = ' \n'
[204]:
line.strip()
[204]:
''
File I/O¶
Question: do binary files also iterate line by line?
[185]:
f = open('/etc/passwd', 'br')
[186]:
for line in f:
print(line)
b'root:x:0:0:root:/root:/bin/bash\n'
b'bin:x:1:1:bin:/bin:/sbin/nologin\n'
b'daemon:x:2:2:daemon:/sbin:/sbin/nologin\n'
b'adm:x:3:4:adm:/var/adm:/sbin/nologin\n'
b'lp:x:4:7:lp:/var/spool/lpd:/sbin/nologin\n'
b'sync:x:5:0:sync:/sbin:/bin/sync\n'
b'shutdown:x:6:0:shutdown:/sbin:/sbin/shutdown\n'
b'halt:x:7:0:halt:/sbin:/sbin/halt\n'
b'mail:x:8:12:mail:/var/spool/mail:/sbin/nologin\n'
b'operator:x:11:0:operator:/root:/sbin/nologin\n'
b'games:x:12:100:games:/usr/games:/sbin/nologin\n'
b'ftp:x:14:50:FTP User:/var/ftp:/sbin/nologin\n'
b'nobody:x:65534:65534:Kernel Overflow User:/:/sbin/nologin\n'
b'apache:x:48:48:Apache:/usr/share/httpd:/sbin/nologin\n'
b'systemd-network:x:192:192:systemd Network Management:/:/sbin/nologin\n'
b'systemd-coredump:x:999:997:systemd Core Dumper:/:/sbin/nologin\n'
b'systemd-resolve:x:193:193:systemd Resolver:/:/sbin/nologin\n'
b'systemd-oom:x:998:996:systemd Userspace OOM Killer:/:/sbin/nologin\n'
b'systemd-timesync:x:997:995:systemd Time Synchronization:/:/sbin/nologin\n'
b'tss:x:59:59:Account used for TPM access:/dev/null:/sbin/nologin\n'
b'dbus:x:81:81:System message bus:/:/sbin/nologin\n'
b'polkitd:x:996:994:User for polkitd:/:/sbin/nologin\n'
b'avahi:x:70:70:Avahi mDNS/DNS-SD Stack:/var/run/avahi-daemon:/sbin/nologin\n'
b'unbound:x:995:992:Unbound DNS resolver:/etc/unbound:/sbin/nologin\n'
b'dnsmasq:x:994:991:Dnsmasq DHCP and DNS server:/var/lib/dnsmasq:/sbin/nologin\n'
b'nm-openconnect:x:993:989:NetworkManager user for OpenConnect:/:/sbin/nologin\n'
b'usbmuxd:x:113:113:usbmuxd user:/:/sbin/nologin\n'
b'gluster:x:992:988:GlusterFS daemons:/run/gluster:/sbin/nologin\n'
b'rtkit:x:172:172:RealtimeKit:/proc:/sbin/nologin\n'
b'pipewire:x:991:987:PipeWire System Daemon:/var/run/pipewire:/sbin/nologin\n'
b'geoclue:x:990:986:User for geoclue:/var/lib/geoclue:/sbin/nologin\n'
b'chrony:x:989:984::/var/lib/chrony:/sbin/nologin\n'
b'saslauth:x:988:76:Saslauthd user:/run/saslauthd:/sbin/nologin\n'
b'radvd:x:75:75:radvd user:/:/sbin/nologin\n'
b'rpc:x:32:32:Rpcbind Daemon:/var/lib/rpcbind:/sbin/nologin\n'
b'qemu:x:107:107:qemu user:/:/sbin/nologin\n'
b'openvpn:x:987:982:OpenVPN:/etc/openvpn:/sbin/nologin\n'
b'nm-openvpn:x:986:981:Default user for running openvpn spawned by NetworkManager:/:/sbin/nologin\n'
b'colord:x:985:980:User for colord:/var/lib/colord:/sbin/nologin\n'
b'rpcuser:x:29:29:RPC Service User:/var/lib/nfs:/sbin/nologin\n'
b'abrt:x:173:173::/etc/abrt:/sbin/nologin\n'
b'flatpak:x:984:979:User for flatpak system helper:/:/sbin/nologin\n'
b'gdm:x:42:42::/var/lib/gdm:/sbin/nologin\n'
b'gnome-initial-setup:x:983:978::/run/gnome-initial-setup/:/sbin/nologin\n'
b'vboxadd:x:982:1::/var/run/vboxadd:/sbin/nologin\n'
b'sshd:x:74:74:Privilege-separated SSH:/usr/share/empty.sshd:/sbin/nologin\n'
b'tcpdump:x:72:72::/:/sbin/nologin\n'
b'jfasch:x:1000:1000:Joerg Faschingbauer:/home/jfasch:/bin/bash\n'
b'mosquitto:x:981:974:Mosquitto Broker:/etc/mosquitto:/sbin/nologin\n'
b'someone-else:x:1001:1001::/home/someone-else:/bin/bash\n'
Tuples Containing Only One Element¶
[205]:
t = (1, 2, 3)
type(t)
[205]:
tuple
[206]:
t
[206]:
(1, 2, 3)
[207]:
t = (666)
type(t)
[207]:
int
[208]:
t
[208]:
666
[209]:
condition = True
[214]:
if (condition):
print('ahja')
ahja
[211]:
t = ()
type(t)
[211]:
tuple
[212]:
bool(t)
[212]:
False
[213]:
t = (1,2,3)
bool(t)
[213]:
True
[215]:
t = (666,)
type(t)
[215]:
tuple
Iterables, Lists, and the itertools
Modules¶
[219]:
values = [
24000,
24500,
25000,
25500,
26000,
26500,
]
[221]:
for element in values:
print(element)
24000
24500
25000
25500
26000
26500
[222]:
it = iter(values)
type(it)
[222]:
list_iterator
[223]:
next(it)
[223]:
24000
[224]:
next(it)
[224]:
24500
[225]:
next(it)
[225]:
25000
[226]:
next(it)
[226]:
25500
[227]:
next(it)
[227]:
26000
[228]:
next(it)
[228]:
26500
[230]:
try:
next(it)
except Exception as e:
print(type(e), e)
<class 'StopIteration'>
itertools.chain()
¶
[232]:
values
[232]:
[24000, 24500, 25000, 25500, 26000, 26500]
[233]:
import itertools
[234]:
for element in itertools.chain(values, values):
print(element)
24000
24500
25000
25500
26000
26500
24000
24500
25000
25500
26000
26500
Destructor?¶
[235]:
class Whatever:
def __init__(self):
print('init')
def __del__(self):
print('del')
[236]:
whe = Whatever()
init
[237]:
del whe
del
Tuple Unpacking on Dictionaries¶
[238]:
d = {
'ID': '5',
'Firstname': 'Joerg',
'Lastname': 'Faschingbauer',
'Birth': '19.6.1966',
}
[240]:
id, firstname, lastname, birth = d
[241]:
id, firstname, lastname, birth
[241]:
('ID', 'Firstname', 'Lastname', 'Birth')
[242]:
for k in d:
print(k)
ID
Firstname
Lastname
Birth
[243]:
id, firstname, lastname, birth = d.values()
[244]:
id, firstname, lastname, birth
[244]:
('5', 'Joerg', 'Faschingbauer', '19.6.1966')
subprocess
¶
[249]:
import subprocess
[250]:
subprocess.run(['ls', '-l', '-a'])
total 144
drwxrwxr-x. 1 jfasch jfasch 148 May 5 15:37 .
drwxrwxr-x. 1 jfasch jfasch 816 Apr 28 11:20 ..
-rw-rw-r--. 1 jfasch jfasch 13397 May 5 11:21 index.rst
-rw-rw-r--. 1 jfasch jfasch 6716 Apr 28 11:13 index.rst.~1~
drwxrwxr-x. 1 jfasch jfasch 50 May 2 08:40 .ipynb_checkpoints
-rw-rw-r--. 1 jfasch jfasch 116985 May 5 15:37 notebook.ipynb
-rw-rw-r--. 1 jfasch jfasch 112 Apr 28 11:18 notebook-wrapper.rst
[250]:
CompletedProcess(args=['ls', '-l', '-a'], returncode=0)
[261]:
completed = subprocess.run(['ls', '-l', '-a'], capture_output=True)
[253]:
completed.returncode
[253]:
0
[262]:
completed.stdout
[262]:
b'total 148\ndrwxrwxr-x. 1 jfasch jfasch 148 May 5 15:47 .\ndrwxrwxr-x. 1 jfasch jfasch 816 Apr 28 11:20 ..\n-rw-rw-r--. 1 jfasch jfasch 13397 May 5 11:21 index.rst\n-rw-rw-r--. 1 jfasch jfasch 6716 Apr 28 11:13 index.rst.~1~\ndrwxrwxr-x. 1 jfasch jfasch 50 May 2 08:40 .ipynb_checkpoints\n-rw-rw-r--. 1 jfasch jfasch 120725 May 5 15:47 notebook.ipynb\n-rw-rw-r--. 1 jfasch jfasch 112 Apr 28 11:18 notebook-wrapper.rst\n'
[263]:
completed.stderr
[263]:
b''
[256]:
completed.stdout.split(b'\n')
[256]:
[b'total 144',
b'drwxrwxr-x. 1 jfasch jfasch 148 May 5 15:38 .',
b'drwxrwxr-x. 1 jfasch jfasch 816 Apr 28 11:20 ..',
b'-rw-rw-r--. 1 jfasch jfasch 13397 May 5 11:21 index.rst',
b'-rw-rw-r--. 1 jfasch jfasch 6716 Apr 28 11:13 index.rst.~1~',
b'drwxrwxr-x. 1 jfasch jfasch 50 May 2 08:40 .ipynb_checkpoints',
b'-rw-rw-r--. 1 jfasch jfasch 118110 May 5 15:38 notebook.ipynb',
b'-rw-rw-r--. 1 jfasch jfasch 112 Apr 28 11:18 notebook-wrapper.rst',
b'']
[257]:
subprocess.run(['wc', '-l'])
0
[257]:
CompletedProcess(args=['wc', '-l'], returncode=0)
[264]:
%matplotlib inline
[266]:
import matplotlib.pyplot as plt
[267]:
x = [1, 2, 3, 4]
y = [i**2 for i in x]
[268]:
plt.plot(x, y)
[268]:
[<matplotlib.lines.Line2D at 0x7fe3a392aa30>]
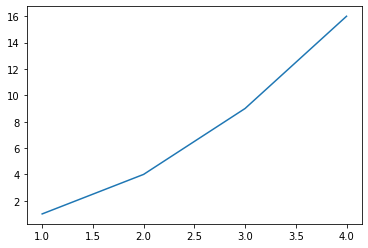