Initial Terminology¶
Sequence¶
Sequence is an entire class of data types which have a few things in commmon: elements have positions, elements are ordered.
[1]:
l = [1, 2, 3, 'vier'] # list
l[0]
[2]:
l[1]
[2]:
2
[3]:
l[3]
[3]:
'vier'
[4]:
s = 'Hello World' # str
s[0]
[4]:
'H'
[5]:
s[6]
[5]:
'W'
Variables¶
[6]:
a = 42
type(a)
[6]:
int
[7]:
a = 'hello'
type(a)
[7]:
str
[8]:
a = 42.345
type(a)
[8]:
float
[9]:
a = [1, 2, 3, 'vier']
type(a)
[9]:
list
Datatypes¶
[10]:
i = 666
Highest natively representable number:
[11]:
2**64 - 1
[11]:
18446744073709551615
[12]:
2**64
[12]:
18446744073709551616
[13]:
2**100
[13]:
1267650600228229401496703205376
[14]:
2**1000
[14]:
10715086071862673209484250490600018105614048117055336074437503883703510511249361224931983788156958581275946729175531468251871452856923140435984577574698574803934567774824230985421074605062371141877954182153046474983581941267398767559165543946077062914571196477686542167660429831652624386837205668069376
Modulo Operator: %
[15]:
5%2
[15]:
1
[16]:
6%4
[16]:
2
[17]:
6%3
[17]:
0
Negation vs. Subtraction
[18]:
a, b = 2, 3
[19]:
b-a
[19]:
1
[20]:
-b
[20]:
-3
Strings¶
[4]:
hour = 9
sex = 'm'
if hour >= 9 and hour < 11:
greeting = 'Good Morning'
# ...
if sex == 'm':
greeting += ', Mr.'
# ...
print(greeting)
Good Morning, Mr.
Boolean¶
[5]:
if True:
print('jo')
jo
[14]:
s = 'x'
if len(s) == 0:
print('string is empty')
else:
print('string is not empty')
string is not empty
Strings evaluate to boolean …
[17]:
s = 'x'
[18]:
if s:
print('string is not empty')
else:
print('string is empty')
string is not empty
[20]:
if not s:
print('string is empty')
else:
print('string is not empty')
string is not empty
[15]:
sex = 'x'
if sex == 'm' or 'M':
print('ja')
ja
[16]:
if 'M':
print('jo')
else:
print('na')
jo
Compound Datatypes¶
List (mutable)¶
[21]:
l = [1,2,3,'vier', ['noch', 1, 'liste']]
l
[21]:
[1, 2, 3, 'vier', ['noch', 1, 'liste']]
[22]:
l[3]
[22]:
'vier'
[23]:
l.append(6.0)
[24]:
l
[24]:
[1, 2, 3, 'vier', ['noch', 1, 'liste'], 6.0]
[25]:
l.extend([7, 8, 9])
[26]:
l
[26]:
[1, 2, 3, 'vier', ['noch', 1, 'liste'], 6.0, 7, 8, 9]
[27]:
del l[3]
l
[27]:
[1, 2, 3, ['noch', 1, 'liste'], 6.0, 7, 8, 9]
‘+’ leaves operands unmodified:
[28]:
l + [10, 'elf']
[28]:
[1, 2, 3, ['noch', 1, 'liste'], 6.0, 7, 8, 9, 10, 'elf']
[29]:
l
[29]:
[1, 2, 3, ['noch', 1, 'liste'], 6.0, 7, 8, 9]
Tuple (immutable)¶
[30]:
t = (1, 2, 3, 'vier')
t
[30]:
(1, 2, 3, 'vier')
[31]:
t[1]
[31]:
2
Item deletion not possible (for example)
[32]:
try:
del t[1]
except TypeError:
pass
[33]:
try:
t.append(666)
except AttributeError:
pass
Dictionary¶
[34]:
table = {
'zero': 0,
'one': 1,
# ...
'nine': 9,
}
[35]:
table['nine']
[35]:
9
[36]:
try:
table['six']
except KeyError as e:
print(e)
'six'
[37]:
table['six'] = 6
[39]:
table['six']
[39]:
6
[40]:
del table['six']
[41]:
'six' in table
[41]:
False
[42]:
'one' in table
[42]:
True
Set¶
[43]:
s = { 1, 2, 3, 'vier' }
s
[43]:
{1, 2, 3, 'vier'}
[44]:
s.add(5.0)
[45]:
s
[45]:
{1, 2, 3, 5.0, 'vier'}
[46]:
5.0 in s
[46]:
True
[47]:
6 not in s
[47]:
True
[49]:
not 6 in s
[49]:
True
[50]:
{ 1, 2, 3} | { 1, 2, 4}
[50]:
{1, 2, 3, 4}
[51]:
{ 1, 2, 3} & { 1, 2, 4}
[51]:
{1, 2}
References, (Im)mutability¶
[52]:
l1 = [1, 2, 3, 'vier']
l2 = l1
[53]:
l1
[53]:
[1, 2, 3, 'vier']
[54]:
l2
[54]:
[1, 2, 3, 'vier']
[56]:
id(l1)
[56]:
140576769461632
[57]:
id(l2)
[57]:
140576769461632
[58]:
l1.append(5.0)
l1
[58]:
[1, 2, 3, 'vier', 5.0]
[59]:
l2
[59]:
[1, 2, 3, 'vier', 5.0]
Iteration, for
, and range()
¶
[22]:
l = ['eins', 'zwei', 'drei', 'vier']
for element in l:
print(element)
eins
zwei
drei
vier
[24]:
l = [0, 1, 2, 3]
for element in l:
print(element)
0
1
2
3
[36]:
for element in range(0, 4):
print(element)
0
1
2
3
Iterator Protocol (not so important for beginners)¶
[26]:
r = range(0,4)
type(r)
[26]:
range
[28]:
iterator = iter(r)
[29]:
next(iterator)
[29]:
0
[30]:
next(iterator)
[30]:
1
[31]:
next(iterator)
[31]:
2
[32]:
next(iterator)
[32]:
3
[34]:
try:
next(iterator)
except StopIteration:
pass
Iteration, and Compound Datatypes¶
List and Tuple¶
[37]:
l = [1, 2, 3, 'vier']
for element in l:
print(element)
1
2
3
vier
[38]:
t = (1, 2, 3, 'vier')
for element in t:
print(element)
1
2
3
vier
Set¶
[40]:
s = {1, 2, 3, 'vier'}
for element in s:
print(element)
1
2
3
vier
Order ist not guaranteed:
[41]:
s.add(5.0)
for element in s:
print(element)
1
2
3
5.0
vier
Dictionary¶
[42]:
table = {
'one': 1,
'two': 2,
'three': 3,
}
Dictionary iteration is simplicit key iteration
[43]:
for element in table:
print(element)
one
two
three
Explicit is better than implicit:
[46]:
for key in table.keys():
print(key)
one
two
three
How do I iterate over values?
[49]:
for value in table.values():
print(value)
1
2
3
[51]:
for item in table.items():
print(item)
('one', 1)
('two', 2)
('three', 3)
[53]:
for item in table.items():
key = item[0]
value = item[1]
print('key:', key, ', value:', value)
key: one , value: 1
key: two , value: 2
key: three , value: 3
Tuple unpacking:
[54]:
for key, value in table.items():
print('key:', key, ', value:', value)
key: one , value: 1
key: two , value: 2
key: three , value: 3
Functions¶
Calculate maximum of two variables …
[55]:
a = 100
b = 200
[56]:
if a < b:
print(b)
else:
print(a)
200
[57]:
def maximum(lhs, rhs):
if lhs < rhs:
return rhs
else:
return lhs
[59]:
m = maximum(a, b)
print(m)
200
Only integers? And strings?
[60]:
'abc' < 'def'
[60]:
True
[62]:
maximum('abc', 'def')
[62]:
'def'
[65]:
try:
maximum(42, '666')
except TypeError as e:
print(e)
'<' not supported between instances of 'int' and 'str'
matplotlib
¶
[68]:
%matplotlib inline
[69]:
import matplotlib.pyplot as plt
[129]:
plt.plot([0, 1, 2, 3, 4, 5], [3, 4, 7, 15, 30, 45])
plt.plot([0, 1, 2, 3, 4, 5], [3, 4, 5, 6, 7, 8])
plt
[129]:
<module 'matplotlib.pyplot' from '/home/jfasch/venv/homepage/lib64/python3.9/site-packages/matplotlib/pyplot.py'>
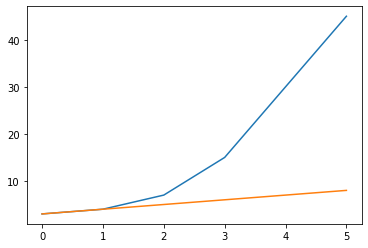
File I/O, and Strings¶
[75]:
f = open('inputfile.csv')
for line in f:
print(line)
0;200;0.5
1;202;10
2;220;15
3;300;40
4;450;80
5;600;150
6;1100;3000
Hm. We want to split each line into three elements, and store those columns in three lists: time
, pressure
, and temperature
. See the extra program on Github.
[ ]:
Strings¶
Quoting?¶
[77]:
s = 'hello world'
s
[77]:
'hello world'
[79]:
s = "hello world"
s
[79]:
'hello world'
[83]:
s = 'das ist ein single-quote: \''
s
[83]:
"das ist ein single-quote: '"
[84]:
s = "das ist ein single-quote: '"
s
[84]:
"das ist ein single-quote: '"
Raw strings: Windows path names¶
[89]:
filename = 'C:\some\\name.txt' # WTF?
print(filename)
C:\some\name.txt
[91]:
filename = r'C:\some\name.txt'
print(filename)
C:\some\name.txt
Multiline Strings¶
[93]:
paragraph = 'Eine Zeile\nNoch eine Zeile\nUnd noch viel mehr\n'
print(paragraph)
Eine Zeile
Noch eine Zeile
Und noch viel mehr
[95]:
paragraph = '''Eine Zeile
Noch eine Zeile
Und noch viel mehr
'''
print(paragraph)
Eine Zeile
Noch eine Zeile
Und noch viel mehr
Commonly used to write docstrings:
[96]:
# this highly sophisticated algorithm determines
# the maximum blah blah
def maximum(lhs, rhs):
if lhs < rhs:
return rhs
else:
return lhs
[100]:
def maximum(lhs, rhs):
'''this highly sophisticated algorithm determines
the maximum blah blah
'''
if lhs < rhs:
return rhs
else:
return lhs
[102]:
maximum.__doc__
[102]:
'this highly sophisticated algorithm determines \n the maximum blah blah\n '
[104]:
help(maximum)
Help on function maximum in module __main__:
maximum(lhs, rhs)
this highly sophisticated algorithm determines
the maximum blah blah
[105]:
import sys
[106]:
help(sys)
Help on built-in module sys:
NAME
sys
MODULE REFERENCE
https://docs.python.org/3.9/library/sys
The following documentation is automatically generated from the Python
source files. It may be incomplete, incorrect or include features that
are considered implementation detail and may vary between Python
implementations. When in doubt, consult the module reference at the
location listed above.
DESCRIPTION
This module provides access to some objects used or maintained by the
interpreter and to functions that interact strongly with the interpreter.
Dynamic objects:
argv -- command line arguments; argv[0] is the script pathname if known
path -- module search path; path[0] is the script directory, else ''
modules -- dictionary of loaded modules
displayhook -- called to show results in an interactive session
excepthook -- called to handle any uncaught exception other than SystemExit
To customize printing in an interactive session or to install a custom
top-level exception handler, assign other functions to replace these.
stdin -- standard input file object; used by input()
stdout -- standard output file object; used by print()
stderr -- standard error object; used for error messages
By assigning other file objects (or objects that behave like files)
to these, it is possible to redirect all of the interpreter's I/O.
last_type -- type of last uncaught exception
last_value -- value of last uncaught exception
last_traceback -- traceback of last uncaught exception
These three are only available in an interactive session after a
traceback has been printed.
Static objects:
builtin_module_names -- tuple of module names built into this interpreter
copyright -- copyright notice pertaining to this interpreter
exec_prefix -- prefix used to find the machine-specific Python library
executable -- absolute path of the executable binary of the Python interpreter
float_info -- a named tuple with information about the float implementation.
float_repr_style -- string indicating the style of repr() output for floats
hash_info -- a named tuple with information about the hash algorithm.
hexversion -- version information encoded as a single integer
implementation -- Python implementation information.
int_info -- a named tuple with information about the int implementation.
maxsize -- the largest supported length of containers.
maxunicode -- the value of the largest Unicode code point
platform -- platform identifier
prefix -- prefix used to find the Python library
thread_info -- a named tuple with information about the thread implementation.
version -- the version of this interpreter as a string
version_info -- version information as a named tuple
__stdin__ -- the original stdin; don't touch!
__stdout__ -- the original stdout; don't touch!
__stderr__ -- the original stderr; don't touch!
__displayhook__ -- the original displayhook; don't touch!
__excepthook__ -- the original excepthook; don't touch!
Functions:
displayhook() -- print an object to the screen, and save it in builtins._
excepthook() -- print an exception and its traceback to sys.stderr
exc_info() -- return thread-safe information about the current exception
exit() -- exit the interpreter by raising SystemExit
getdlopenflags() -- returns flags to be used for dlopen() calls
getprofile() -- get the global profiling function
getrefcount() -- return the reference count for an object (plus one :-)
getrecursionlimit() -- return the max recursion depth for the interpreter
getsizeof() -- return the size of an object in bytes
gettrace() -- get the global debug tracing function
setdlopenflags() -- set the flags to be used for dlopen() calls
setprofile() -- set the global profiling function
setrecursionlimit() -- set the max recursion depth for the interpreter
settrace() -- set the global debug tracing function
FUNCTIONS
__breakpointhook__ = breakpointhook(...)
breakpointhook(*args, **kws)
This hook function is called by built-in breakpoint().
__displayhook__ = displayhook(object, /)
Print an object to sys.stdout and also save it in builtins._
__excepthook__ = excepthook(exctype, value, traceback, /)
Handle an exception by displaying it with a traceback on sys.stderr.
__unraisablehook__ = unraisablehook(unraisable, /)
Handle an unraisable exception.
The unraisable argument has the following attributes:
* exc_type: Exception type.
* exc_value: Exception value, can be None.
* exc_traceback: Exception traceback, can be None.
* err_msg: Error message, can be None.
* object: Object causing the exception, can be None.
addaudithook(hook)
Adds a new audit hook callback.
audit(...)
audit(event, *args)
Passes the event to any audit hooks that are attached.
breakpointhook(...)
breakpointhook(*args, **kws)
This hook function is called by built-in breakpoint().
call_tracing(func, args, /)
Call func(*args), while tracing is enabled.
The tracing state is saved, and restored afterwards. This is intended
to be called from a debugger from a checkpoint, to recursively debug
some other code.
exc_info()
Return current exception information: (type, value, traceback).
Return information about the most recent exception caught by an except
clause in the current stack frame or in an older stack frame.
exit(status=None, /)
Exit the interpreter by raising SystemExit(status).
If the status is omitted or None, it defaults to zero (i.e., success).
If the status is an integer, it will be used as the system exit status.
If it is another kind of object, it will be printed and the system
exit status will be one (i.e., failure).
get_asyncgen_hooks()
Return the installed asynchronous generators hooks.
This returns a namedtuple of the form (firstiter, finalizer).
get_coroutine_origin_tracking_depth()
Check status of origin tracking for coroutine objects in this thread.
getallocatedblocks()
Return the number of memory blocks currently allocated.
getdefaultencoding()
Return the current default encoding used by the Unicode implementation.
getdlopenflags()
Return the current value of the flags that are used for dlopen calls.
The flag constants are defined in the os module.
getfilesystemencodeerrors()
Return the error mode used Unicode to OS filename conversion.
getfilesystemencoding()
Return the encoding used to convert Unicode filenames to OS filenames.
getprofile()
Return the profiling function set with sys.setprofile.
See the profiler chapter in the library manual.
getrecursionlimit()
Return the current value of the recursion limit.
The recursion limit is the maximum depth of the Python interpreter
stack. This limit prevents infinite recursion from causing an overflow
of the C stack and crashing Python.
getrefcount(object, /)
Return the reference count of object.
The count returned is generally one higher than you might expect,
because it includes the (temporary) reference as an argument to
getrefcount().
getsizeof(...)
getsizeof(object [, default]) -> int
Return the size of object in bytes.
getswitchinterval()
Return the current thread switch interval; see sys.setswitchinterval().
gettrace()
Return the global debug tracing function set with sys.settrace.
See the debugger chapter in the library manual.
intern(string, /)
``Intern'' the given string.
This enters the string in the (global) table of interned strings whose
purpose is to speed up dictionary lookups. Return the string itself or
the previously interned string object with the same value.
is_finalizing()
Return True if Python is exiting.
set_asyncgen_hooks(...)
set_asyncgen_hooks(* [, firstiter] [, finalizer])
Set a finalizer for async generators objects.
set_coroutine_origin_tracking_depth(depth)
Enable or disable origin tracking for coroutine objects in this thread.
Coroutine objects will track 'depth' frames of traceback information
about where they came from, available in their cr_origin attribute.
Set a depth of 0 to disable.
setdlopenflags(flags, /)
Set the flags used by the interpreter for dlopen calls.
This is used, for example, when the interpreter loads extension
modules. Among other things, this will enable a lazy resolving of
symbols when importing a module, if called as sys.setdlopenflags(0).
To share symbols across extension modules, call as
sys.setdlopenflags(os.RTLD_GLOBAL). Symbolic names for the flag
modules can be found in the os module (RTLD_xxx constants, e.g.
os.RTLD_LAZY).
setprofile(...)
setprofile(function)
Set the profiling function. It will be called on each function call
and return. See the profiler chapter in the library manual.
setrecursionlimit(limit, /)
Set the maximum depth of the Python interpreter stack to n.
This limit prevents infinite recursion from causing an overflow of the C
stack and crashing Python. The highest possible limit is platform-
dependent.
setswitchinterval(interval, /)
Set the ideal thread switching delay inside the Python interpreter.
The actual frequency of switching threads can be lower if the
interpreter executes long sequences of uninterruptible code
(this is implementation-specific and workload-dependent).
The parameter must represent the desired switching delay in seconds
A typical value is 0.005 (5 milliseconds).
settrace(...)
settrace(function)
Set the global debug tracing function. It will be called on each
function call. See the debugger chapter in the library manual.
unraisablehook(unraisable, /)
Handle an unraisable exception.
The unraisable argument has the following attributes:
* exc_type: Exception type.
* exc_value: Exception value, can be None.
* exc_traceback: Exception traceback, can be None.
* err_msg: Error message, can be None.
* object: Object causing the exception, can be None.
DATA
__stderr__ = <_io.TextIOWrapper name='<stderr>' mode='w' encoding='utf...
__stdin__ = <_io.TextIOWrapper name='<stdin>' mode='r' encoding='utf-8...
__stdout__ = <_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf...
abiflags = ''
api_version = 1013
argv = ['/home/jfasch/venv/homepage/lib64/python3.9/site-packages/ipyk...
base_exec_prefix = '/usr'
base_prefix = '/usr'
builtin_module_names = ('_abc', '_ast', '_codecs', '_collections', '_f...
byteorder = 'little'
copyright = 'Copyright (c) 2001-2021 Python Software Foundati...ematis...
displayhook = <ipykernel.displayhook.ZMQShellDisplayHook object>
dont_write_bytecode = False
exec_prefix = '/home/jfasch/venv/homepage'
executable = '/home/jfasch/venv/homepage/bin/python'
flags = sys.flags(debug=0, inspect=0, interactive=0, opt...ation=1, is...
float_info = sys.float_info(max=1.7976931348623157e+308, max_...epsilo...
float_repr_style = 'short'
hash_info = sys.hash_info(width=64, modulus=2305843009213693...iphash2...
hexversion = 50922736
implementation = namespace(name='cpython', cache_tag='cpython-39'...xv...
int_info = sys.int_info(bits_per_digit=30, sizeof_digit=4)
last_value = SyntaxError('EOL while scanning string literal',...', 1, ...
maxsize = 9223372036854775807
maxunicode = 1114111
meta_path = [<class '_frozen_importlib.BuiltinImporter'>, <class '_fro...
modules = {'IPython': <module 'IPython' from '/home/jfasch/venv/homepa...
path = ['/home/jfasch/work/jfasch-home/trainings/log/detail/2021-08-31...
path_hooks = [<class 'zipimport.zipimporter'>, <function FileFinder.pa...
path_importer_cache = {'/home/jfasch/.ipython': FileFinder('/home/jfas...
platform = 'linux'
platlibdir = 'lib64'
prefix = '/home/jfasch/venv/homepage'
ps1 = 'In : '
ps2 = '...: '
ps3 = 'Out: '
pycache_prefix = None
stderr = <ipykernel.iostream.OutStream object>
stdin = <_io.TextIOWrapper name='<stdin>' mode='r' encoding='utf-8'>
stdout = <ipykernel.iostream.OutStream object>
thread_info = sys.thread_info(name='pthread', lock='semaphore', versio...
version = '3.9.4 (default, Apr 6 2021, 00:00:00) \n[GCC 11.0.1 202103...
version_info = sys.version_info(major=3, minor=9, micro=4, releaseleve...
warnoptions = []
FILE
(built-in)
Miscellaneous String Methods¶
[109]:
'abc'.isalpha()
[109]:
True
[110]:
'abc'.isdigit()
[110]:
False
[112]:
'123'.isdigit()
[112]:
True
[113]:
'abc'.isidentifier()
[113]:
True
[114]:
'123'.isidentifier()
[114]:
False
[115]:
' \r abc \n\t'.strip()
[115]:
'abc'
[116]:
' \r abc \n\t'.rstrip()
[116]:
' \r abc'
[117]:
'mississippi'.find('ss')
[117]:
2
[118]:
'mississippi'.find('xx')
[118]:
-1
[120]:
'mississippi'.count('i')
[120]:
4
[122]:
'mississippi'.count('pp')
[122]:
1
[123]:
list_of_strings = ['eins', 'zwei', 'drei']
'-'.join(list_of_strings)
[123]:
'eins-zwei-drei'
[124]:
line = '0.5;500;5000'
line.split(';')
[124]:
['0.5', '500', '5000']
Tuple unpacking
[125]:
time, pressure, temperature = line.split(';')
[128]:
print('Time:', time, ', Pressure:', pressure, ', Temperature:', temperature)
Time: 0.5 , Pressure: 500 , Temperature: 5000